Manual¶
Information available about Tor from its manual. This provides three methods of getting this information...
- from_cache() provides manual content bundled with Stem. This is the fastest and most reliable method but only as up-to-date as Stem's release.
- from_man() reads Tor's local man page for information about it.
- from_remote() fetches the latest manual information remotely. This is the slowest and least reliable method but provides the most recent information about Tor.
Manual information includes arguments, signals, and probably most usefully the torrc configuration options. For example, say we want a little script that told us what our torrc options do...
from stem.manual import Manual
from stem.util import term
try:
print("Downloading tor's manual information, please wait...")
manual = Manual.from_remote()
print(" done\n")
except IOError as exc:
print(" unsuccessful (%s), using information provided with stem\n" % exc)
manual = Manual.from_cache() # fall back to our bundled manual information
print('Which tor configuration would you like to learn about? (press ctrl+c to quit)\n')
try:
while True:
requested_option = raw_input('> ').strip()
if requested_option:
if requested_option in manual.config_options:
option = manual.config_options[requested_option]
print(term.format('%s %s' % (option.name, option.usage), term.Color.GREEN, term.Attr.BOLD))
print(term.format(option.summary, term.Color.GREEN)) # brief description provided by stem
print(term.format('\nFull Description:\n', term.Color.GREEN, term.Attr.BOLD))
print(term.format(option.description + '\n', term.Color.GREEN))
else:
print(term.format("Sorry, we don't have any information about %s. Are you sure it's an option?" % requested_option, term.Color.RED))
except KeyboardInterrupt:
pass # user pressed ctrl+c
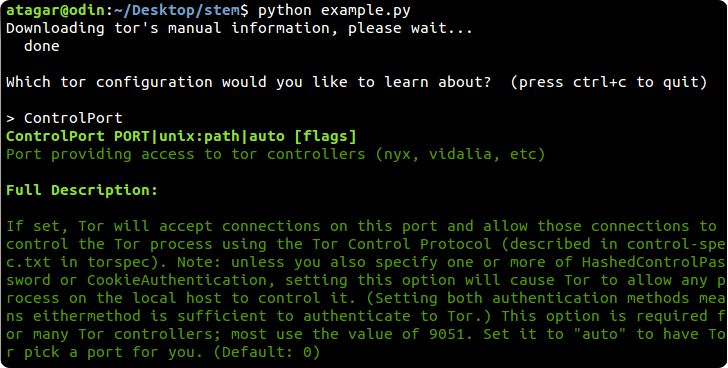
Module Overview:
query - performs a query on our cached sqlite manual information
is_important - Indicates if a configuration option is of particularly common importance.
download_man_page - Downloads tor's latest man page.
Manual - Information about Tor available from its manual.
| |- from_cache - Provides manual information cached with Stem.
| |- from_man - Retrieves manual information from its man page.
| +- from_remote - Retrieves manual information remotely from tor's latest manual.
|
+- save - writes the manual contents to a given location
New in version 1.5.0.
- exception stem.manual.SchemaMismatch(message, database_schema, library_schema)[source]¶
Bases: exceptions.IOError
Database schema doesn't match what Stem supports.
New in version 1.6.0.
Variables: - database_schema (int) -- schema of the database
- supported_schemas (tuple) -- schemas library supports
- stem.manual.query(query, *param)[source]¶
Performs the given query on our sqlite manual cache. This database should be treated as being read-only. File permissions generally enforce this, and in the future will be enforced by this function as well.
>>> import stem.manual >>> print(stem.manual.query('SELECT description FROM torrc WHERE key=?', 'CONTROLSOCKET').fetchone()[0]) Like ControlPort, but listens on a Unix domain socket, rather than a TCP socket. 0 disables ControlSocket. (Unix and Unix-like systems only.) (Default: 0)
New in version 1.6.0.
Parameters: - query (str) -- query to run on the cache
- param (list) -- query parameters
Returns: sqlite3.Cursor with the query results
Raises : - ImportError if the sqlite3 module is unavailable
- sqlite3.OperationalError if query fails
- class stem.manual.ConfigOption(name, category='Unknown', usage='', summary='', description='')[source]¶
Bases: object
Tor configuration attribute found in its torrc.
Variables: - name (str) -- name of the configuration option
- category (stem.manual.Category) -- category the config option was listed under, this is Category.UNKNOWN if we didn't recognize the category
- usage (str) -- arguments accepted by the option
- summary (str) -- brief description of what the option does
- description (str) -- longer manual description with details
- stem.manual.is_important(option)[source]¶
Indicates if a configuration option of particularly common importance or not.
Parameters: option (str) -- tor configuration option to check Returns: bool that's True if this is an important option and False otherwise
- stem.manual.download_man_page(path=None, file_handle=None, url='https://gitweb.torproject.org/tor.git/plain/doc/tor.1.txt', timeout=20)[source]¶
Downloads tor's latest man page from gitweb.torproject.org. This method is both slow and unreliable - please see the warnings on from_remote().
Parameters: - path (str) -- path to save tor's man page to
- file_handle (file) -- file handler to save tor's man page to
- url (str) -- url to download tor's asciidoc manual from
- timeout (int) -- seconds to wait before timing out the request
Raises : IOError if unable to retrieve the manual
- class stem.manual.Manual(name, synopsis, description, commandline_options, signals, files, config_options)[source]¶
Bases: object
Parsed tor man page. Tor makes no guarantees about its man page format so this may not always be compatible. If not you can use the cached manual information stored with Stem.
This does not include every bit of information from the tor manual. For instance, I've excluded the 'THE CONFIGURATION FILE FORMAT' section. If there's a part you'd find useful then file an issue and we can add it.
Variables: - name (str) -- brief description of the tor command
- synopsis (str) -- brief tor command usage
- description (str) -- general description of what tor does
- commandline_options (collections.OrderedDict) -- mapping of commandline arguments to their descripton
- signals (collections.OrderedDict) -- mapping of signals tor accepts to their description
- files (collections.OrderedDict) -- mapping of file paths to their description
- config_options (collections.OrderedDict) -- ConfigOption tuples for tor configuration options
- man_commit (str) -- latest tor commit editing the man page when this information was cached
- stem_commit (str) -- stem commit to cache this manual information
- static from_cache(path=None)[source]¶
Provides manual information cached with Stem. Unlike from_man() and from_remote() this doesn't have any system requirements, and is faster too. Only drawback is that this manual content is only as up to date as the Stem release we're using.
Changed in version 1.6.0: Added support for sqlite cache. Support for Config caches will be dropped in Stem 2.x.
Parameters: path (str) -- cached manual content to read, if not provided this uses the bundled manual information
Returns: Manual with our bundled manual information
Raises : - ImportError if cache is sqlite and the sqlite3 module is unavailable
- IOError if a path was provided and we were unable to read it or the schema is out of date
- static from_man(man_path='tor')[source]¶
Reads and parses a given man page.
On OSX the man command doesn't have an '--encoding' argument so its results may not quite match other platforms. For instance, it normalizes long dashes into '--'.
Parameters: man_path (str) -- path argument for 'man', for example you might want '/path/to/tor/doc/tor.1' to read from tor's git repository Returns: Manual for the system's man page Raises : IOError if unable to retrieve the manual
- static from_remote(timeout=60)[source]¶
Reads and parses the latest tor man page from gitweb.torproject.org. Note that while convenient, this reliance on GitWeb means you should alway call with a fallback, such as...
try: manual = stem.manual.from_remote() except IOError: manual = stem.manual.from_cache()
In addition to our GitWeb dependency this requires 'a2x' which is part of asciidoc and... isn't quick. Personally this takes ~7.41s, breaking down for me as follows...
- 1.67s to download tor.1.txt
- 5.57s to convert the asciidoc to a man page
- 0.17s for stem to read and parse the manual
Parameters: timeout (int) -- seconds to wait before timing out the request Returns: latest Manual available for tor Raises : IOError if unable to retrieve the manual
- save(path)[source]¶
Persists the manual content to a given location.
Changed in version 1.6.0: Added support for sqlite cache. Support for Config caches will be dropped in Stem 2.x.
Parameters: path (str) -- path to save our manual content to
Raises : - ImportError if saving as sqlite and the sqlite3 module is unavailable
- IOError if unsuccessful