Over the River and Through the Wood¶
Hidden services give you a way of providing a service without exposing your address. These services are only accessible through Tor or Tor2web, and useful for a surprising number of things...
- Hosting an anonymized site. This is usually the first thing that comes to mind, and something we'll demonstrate in a sec.
- Providing an endpoint Tor users can reach without exiting the Tor network. This eliminates the risk of an unreliable or malicious exit getting in the way. Great examples of this are Facebook (facebookcorewwwi.onion) and DuckDuckGo (3g2upl4pq6kufc4m.onion).
- Personal services. For instance you can host your home SSH server as a hidden service to prevent eavesdroppers from knowing where you live while traveling abroad.
Tor2web provides a quick and easy way of seeing if your hidden service is working. To use it simply replace the .onion of your address with .tor2web.org...
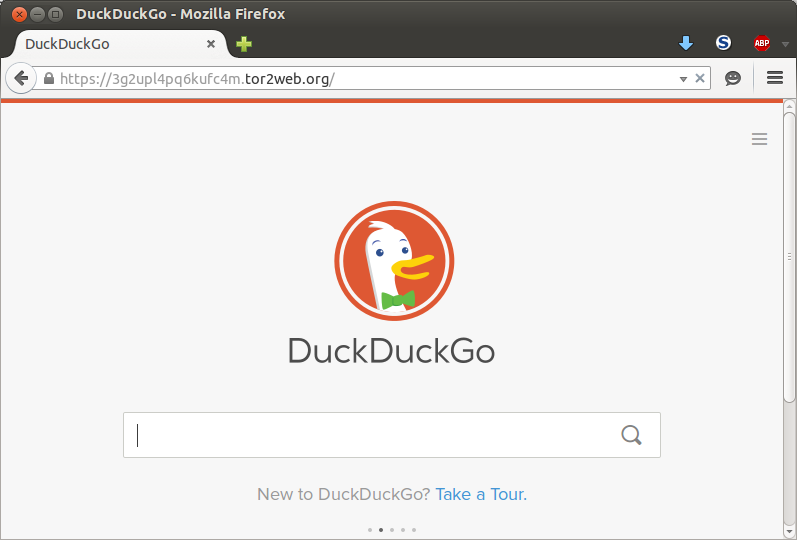
Running a hidden service¶
Hidden services can be configured through your torrc, but Stem also provides some methods to easily work with them...
- create_hidden_service()
- remove_hidden_service()
- get_hidden_service_conf()
- set_hidden_service_conf()
The main threat to your anonymity when running a hidden service is the service itself. Debug information for instance might leak your real address, undermining what Tor provides. This includes the following example, do not rely on it not to leak.
But with that out of the way lets take a look at a simple Flask example based on one by Jordan Wright...
import os
import shutil
from stem.control import Controller
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
return "<h1>Hi Grandma!</h1>"
print(' * Connecting to tor')
with Controller.from_port() as controller:
controller.authenticate()
# All hidden services have a directory on disk. Lets put ours in tor's data
# directory.
hidden_service_dir = os.path.join(controller.get_conf('DataDirectory', '/tmp'), 'hello_world')
# Create a hidden service where visitors of port 80 get redirected to local
# port 5000 (this is where Flask runs by default).
print(" * Creating our hidden service in %s" % hidden_service_dir)
result = controller.create_hidden_service(hidden_service_dir, 80, target_port = 5000)
# The hostname is only available when we can read the hidden service
# directory. This requires us to be running with the same user as tor.
if result.hostname:
print(" * Our service is available at %s, press ctrl+c to quit" % result.hostname)
else:
print(" * Unable to determine our service's hostname, probably due to being unable to read the hidden service directory")
try:
app.run()
finally:
# Shut down the hidden service and clean it off disk. Note that you *don't*
# want to delete the hidden service directory if you'd like to have this
# same *.onion address in the future.
print(" * Shutting down our hidden service")
controller.remove_hidden_service(hidden_service_dir)
shutil.rmtree(hidden_service_dir)
Now if we run this...
% python example.py
* Connecting to tor
* Creating our hidden service in /home/atagar/.tor/hello_world
* Our service is available at uxiuaxejc3sxrb6i.onion, press ctrl+c to quit
* Running on http://127.0.0.1:5000/
127.0.0.1 - - [15/Dec/2014 13:05:43] "GET / HTTP/1.1" 200 -
* Shutting down our hidden service
... we'll have a service we can visit via the Tor Browser Bundle...
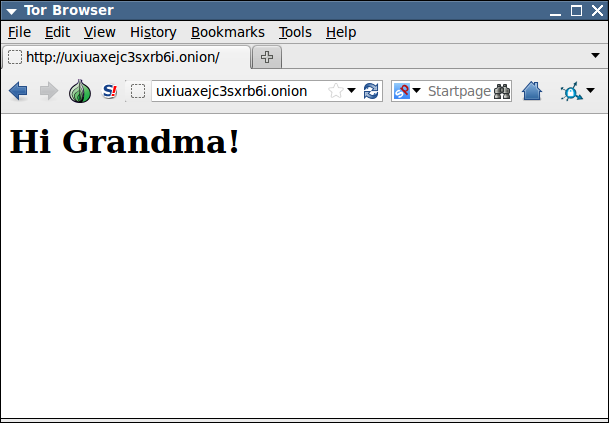
Hidden service authentication¶
Hidden services you create can restrict their access, requiring in essence a password...
>>> from stem.control import Controller
>>> controller = Controller.from_port()
>>> controller.authenticate()
>>> response = controller.create_ephemeral_hidden_service({80: 8080}, await_publication=True, basic_auth={'bob': None, 'alice': None})
>>> response.service_id, response.client_auth
('l3lnorirzn7hrjnw', {'alice': 'I6AMKiay+UkM5MfrvdnF2A', 'bob': 'VLsbrSGyrb5JYEvZmQ3tMg'})
To access this service users simply provide this credential to tor via their torrc or SETCONF prior to visiting it...
>>> controller.set_conf('HidServAuth', 'l3lnorirzn7hrjnw.onion I6AMKiay+UkM5MfrvdnF2A')
Ephemeral hidden services¶
In the above example you may have noticed the note that said...
# The hostname is only available when we can read the hidden service
# directory. This requires us to be running with the same user as tor.
This has been a limitation of hidden services for years. However, as of version 0.2.7.1 Tor offers another style for making services called ephemeral hidden services.
Ephemeral services can only be created through the controller, and only exist as long as your controller is attached unless you provide the detached flag. Controllers can only see their own ephemeral services, and ephemeral services that are detached. In other words, attached ephemeral services can only be managed by their own controller.
Stem provides three methods to work with ephemeral hidden services...
- list_ephemeral_hidden_services()
- create_ephemeral_hidden_service()
- remove_ephemeral_hidden_service()
For example, with a ephemeral service our earlier example becomes as simple as...
from stem.control import Controller
from flask import Flask
app = Flask(__name__)
@app.route('/')
def index():
return "<h1>Hi Grandma!</h1>"
print(' * Connecting to tor')
with Controller.from_port() as controller:
controller.authenticate()
# Create a hidden service where visitors of port 80 get redirected to local
# port 5000 (this is where Flask runs by default).
response = controller.create_ephemeral_hidden_service({80: 5000}, await_publication = True)
print(" * Our service is available at %s.onion, press ctrl+c to quit" % response.service_id)
try:
app.run()
finally:
print(" * Shutting down our hidden service")
Ephemeral hidden services do not touch disk, and as such are easier to work with but require you to persist your service's private key yourself if you want to reuse a '.onion' address...
import os
from stem.control import Controller
key_path = os.path.expanduser('~/my_service_key')
with Controller.from_port() as controller:
controller.authenticate()
if not os.path.exists(key_path):
service = controller.create_ephemeral_hidden_service({80: 5000}, await_publication = True)
print("Started a new hidden service with the address of %s.onion" % service.service_id)
with open(key_path, 'w') as key_file:
key_file.write('%s:%s' % (service.private_key_type, service.private_key))
else:
with open(key_path) as key_file:
key_type, key_content = key_file.read().split(':', 1)
service = controller.create_ephemeral_hidden_service({80: 5000}, key_type = key_type, key_content = key_content, await_publication = True)
print("Resumed %s.onion" % service.service_id)
raw_input('press any key to shut the service down...')
controller.remove_ephemeral_hidden_service(service.service_id)
Hidden service descriptors¶
Like relays, hidden services publish documents about themselves called hidden service descriptors. These contain low level details for establishing connections. Hidden service descriptors are available from the tor process via its get_hidden_service_descriptor() method...
from stem.control import Controller
with Controller.from_port(port = 9051) as controller:
controller.authenticate()
# descriptor of duck-duck-go's hidden service (http://3g2upl4pq6kufc4m.onion)
print(controller.get_hidden_service_descriptor('3g2upl4pq6kufc4m'))
% python print_duck_duck_go_descriptor.py
rendezvous-service-descriptor e5dkwgp6vt7axoozixrbgjymyof7ab6u
version 2
permanent-key
-----BEGIN RSA PUBLIC KEY-----
MIGJAoGBAJ/SzzgrXPxTlFrKVhXh3buCWv2QfcNgncUpDpKouLn3AtPH5Ocys0jE
aZSKdvaiQ62md2gOwj4x61cFNdi05tdQjS+2thHKEm/KsB9BGLSLBNJYY356bupg
I5gQozM65ENelfxYlysBjJ52xSDBd8C4f/p9umdzaaaCmzXG/nhzAgMBAAE=
-----END RSA PUBLIC KEY-----
secret-id-part bmsctib2pzirgo7cltlxdm5fxqcitt5e
publication-time 2015-05-11 20:00:00
protocol-versions 2,3
introduction-points
-----BEGIN MESSAGE-----
aW50cm9kdWN0aW9uLXBvaW50IHZzcm4ycGNtdzNvZ21mNGo3dGpxeHptdml1Y2Rr
NGtpCmlwLWFkZHJlc3MgMTc2LjkuNTkuMTcxCm9uaW9uLXBvcnQgOTAwMQpvbmlv
... etc...
A hidden service's introduction points are a base64 encoded field that's possibly encrypted. These can be decoded (and decrypted if necessary) with the descriptor's introduction_points() method.
from stem.control import Controller
with Controller.from_port(port = 9051) as controller:
controller.authenticate()
desc = controller.get_hidden_service_descriptor('3g2upl4pq6kufc4m')
print("DuckDuckGo's introduction points are...\n")
for introduction_point in desc.introduction_points():
print(' %s:%s => %s' % (introduction_point.address, introduction_point.port, introduction_point.identifier))
% python print_duck_duck_go_introduction_points.py
DuckDuckGo's introduction points are...
176.9.59.171:9001 => vsrn2pcmw3ogmf4j7tjqxzmviucdk4ki
104.131.106.181:9001 => gcl2kpqx5qnkpgxjf6x7ulqncoqj7ghh
188.166.58.218:443 => jeymnbhs2d6l2oib7jjvweavg45m6gju